How to Create a ChatGPT-like Clone With Full Features? A Developer’s Guide for 2025
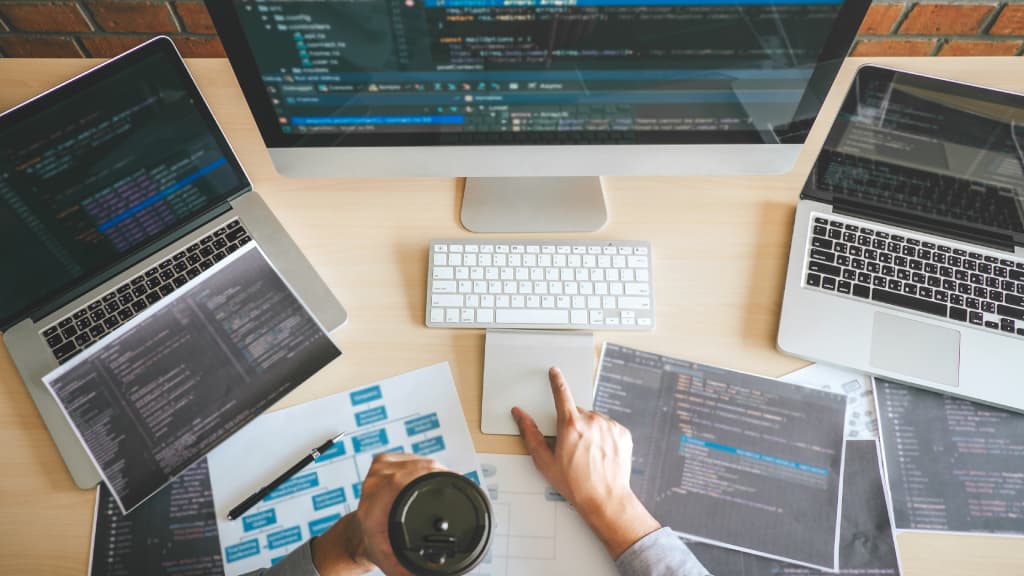
Authoritative Guide for AI Developers | 2025 Edition | GPT-based App Development Artificial Intelligence continues to redefine software capabilities across industries, and conversational AI remains a crown jewel. With the rise of ChatGPT, businesses and developers alike are eager to build similar chat-driven applications, either for internal use, customer engagement, or to launch as commercial SaaS products.
Building a ChatGPT-style application involves multiple development layers, from LLM orchestration and memory management to scalable infrastructure and user-facing UI.
Get to Know ChatGPT’s Core Architecture
At its heart, ChatGPT is powered by transformer-based language models, such as OpenAI’s GPT-4-turbo or GPT-5. These models are trained on massive datasets to understand, generate, and reason through human language.
Key architectural components:
- Frontend UI: Web or mobile chat interface.
- Backend Server: API orchestration, authentication, session handling.
- LLM Inference Engine: Either hosted models (OpenAI, Anthropic) or self-hosted models (LLaMA 3, Mistral, Falcon).
- Memory Layer: For persistent session memory (Redis, PostgreSQL).
- Prompt Engineering Layer: For dynamic prompt construction and context management.
Key Features to Include in a Clone
To create a ChatGPT clone with full parity in 2025, the application must support:
- Natural Language Processing (NLP) with contextual memory
- Conversational threading with session persistence
- Multi-model backend support (GPT, LLaMA, Claude, etc.)
- Voice input/output integration
- Image understanding (multimodal) if targeting GPT-4V feature parity
- Prompt templates & system instructions
- User authentication and permissions
- Chat history and export features
- Custom instructions / personalization
- Usage limits, token tracking, and billing
- Analytics and user behavior tracking
Technology Stack for a ChatGPT-like Clone (2025 Edition)
Here’s a modern, scalable tech stack suitable for production:
Frontend
- React.js / Next.js 14: SSR, SEO, and performance
- Tailwind CSS: Modern, responsive styling
- Framer Motion: Chat animations and transitions
- WebSockets / SSE: Real-time token streaming
- Whisper ASR / Web Speech API: For voice input
Backend
- Node.js with Express / Fastify
- Python (FastAPI) for ML-heavy logic
- LangChain / LlamaIndex: Prompt management, memory, tools
- Redis: Short-term memory, rate-limiting
- PostgreSQL: Persistent chat history, user data
- Auth0 / Clerk: Authentication & session control
LLM Integration
- OpenAI GPT-4 / GPT-4-Turbo APIs
- Self-hosted LLaMA 3 on NVIDIA H100s
- Transformer-based open models (e.g. Mixtral, Claude, Gemini)
Deployment & DevOps
- Docker / Kubernetes
- Vercel (Frontend) + AWS/GCP (Backend)
- GitHub Actions / GitLab CI/CD
- Prometheus + Grafana – Monitoring
- Sentry / LogRocket – Error logging
Step-by-Step Development Process
1. Project Initialization
- Set up mono-repo with TurboRepo
- Scaffold frontend with Next.js App Router
- Backend setup with FastAPI or Express
2. Chat Interface Development
- Token-based message rendering (stream tokens as they arrive)
- Auto-scroll, markdown formatting, code highlighting
- Audio recording with Whisper / browser voice API
3. API Gateway & Message Pipeline
- Receive user message → Preprocess → Send to LLM → Return response
- Use OpenAI API or local inference engine
- Store chat logs in PostgreSQL using UUID thread IDs
4. Prompt Engineering Framework
- Design system prompt templates per use-case
- Add tool calling agents with LangChain
- Build dynamic context injectors using conversation history
5. Session & Memory Management
- Use Redis for short-term memory context
- PostgreSQL for full thread recovery
- Vector DB (e.g., Pinecone, Weaviate) for embedding-based recall
6. User Authentication & Access Control
- JWT-based login/session management
- Role-based access for admin/enterprise accounts
- Stripe integration for usage-based billing
Fine-Tuning and Prompt Engineering
While GPT-4 and Claude are general-purpose, fine-tuning can give your clone niche domain power. Options in 2025:
- LoRA Adapters (Low-Rank Adaptation) for customizing large models
- RAG (Retrieval-Augmented Generation) using vector stores
- Open-Source Tools: Hugging Face PEFT, QLoRA, Axolotl
Prompt Engineering Tips:
- Use structured formatting (system, user, assistant roles)
- Define instruction boundaries (e.g., “You are a friendly legal assistant”)
- Limit response hallucinations with strict guardrails
UI/UX Considerations for AI Chat Interfaces
User trust and usability define adoption.
- Typing indicators & token streaming
- Syntax highlighting for code responses
- Edit & regenerate prompts
- Download transcript as PDF or Markdown
- Dark mode, responsive design, ARIA accessibility
- “Custom GPTs” builder for power users
Performance Optimization and Scaling
Key Areas to Monitor
- Token latency, Measure LLM response time
- Load balancing, Especially for GPU-bound inference
- Session expiration policies
- Rate limiting via Redis or API gateways
Use Kubernetes horizontal autoscaling, GPU autoscaling (NVIDIA Triton), and multi-region deployment for global usage.
Security, Compliance & Privacy Considerations
As AI adoption grows, data governance becomes non-negotiable.
- End-to-end encryption (TLS 1.3)
- PII redaction before LLM processing
- SOC 2, HIPAA, and GDPR compliance frameworks
- Audit logs and permissioned access
- Usage monitoring + abuse detection
Hosting, CI/CD, and DevOps Practices
Tools & Best Practices
- GitHub Actions + Docker Compose for builds
- Helm charts for Kubernetes deployment
- Environment variables via Doppler / HashiCorp Vault
- Automated testing using Cypress (UI) and PyTest (backend)
- Observability using Prometheus, Grafana, Jaeger
Cost Analysis and Maintenance Best Practices
Approximate Costs (monthly for moderate traffic):
Component | Cost Estimate |
OpenAI GPT-4-Turbo API | $100–$500+ |
Vector DB (Pinecone) | $40–$200 |
GPU Inference Server | $300–$1,200 (AWS/GCP) |
DevOps Tooling | $100–$300 |
Developer Time | Variable |
Maintenance Tips
- Regular dependency updates
- Monitor LLM API changes
- Refactor prompts over time
- Add usage analytics to optimize flows
Building a ChatGPT-like Clone in 2025
Stage 1: Planning and Architecture Design
Before writing any code, clearly define:
- The use case (e.g., customer support, content creation, education)
- Target LLM provider (OpenAI, Anthropic, Mistral, or self-hosted)
- Multimodal support needs (images, voice)
- Data security and compliance scope (HIPAA, GDPR, SOC 2)
- Scalability targets (concurrent users, latency budgets)
Deliverables:
a) Technical architecture diagram
b) Feature list and API contracts
c) System and prompt design guidelines
Stage 2: Frontend Development (Chat UI)
Tech Stack
- Next.js 14 with App Router and Server Actions
- Tailwind CSS for utility-first styling
- Framer Motion for smooth message transitions
Features to Implement
1. Message input and rendering
- Stream tokens as they’re generated
- Support Markdown, code blocks, tables
2. Voice input (optional)
Use browser-native APIs or integrate with OpenAI Whisper
3. Session management
- Threaded chat sessions with rename/delete
- Custom instructions per thread
4. State management
- Use Zustand or React Context to store UI states and chat history
5. Chat history viewer
- Load historical messages via lazy loading/pagination
- Auto-scroll & scroll anchor logic
Stage 3: Backend Development (API Orchestration)
Tech Stack
- Node.js (Fastify) or Python (FastAPI)
- PostgreSQL via Prisma or SQLAlchemy
- Redis for short-term memory & queues
Core Modules
1. /chat endpoint
- Accepts user_message, thread_id, and metadata
- Prepares prompt for LLM call
2. Prompt constructor
- Retrieves context (last N messages or embeddings)
- Formats system/user/assistant roles
3. LLM Router
- Selects appropriate model (GPT-4, Claude, LLaMA)
- Sends request via API or local inference server
- Streams response back to frontend (SSE or WebSocket)
4. Persistence layer
- Stores message logs, user data, custom instructions
- Token/usage tracking per session
5. Authentication middleware
- Auth0 / Clerk for secure login
- JWT verification for protected endpoints
Stage 4: Memory, Context, and RAG
Short-Term Memory
- Redis or local store
- Context window trimming (based on token length)
Long-Term Memory
- Vector DBs (e.g., Pinecone, Weaviate, Qdrant)
- Store embeddings using models like text-embedding-3-small
RAG (Retrieval-Augmented Generation)
- User query → search embedding → retrieve relevant docs → inject into context
- Integrate with LangChain’s Retriever chains
Stage 5: Fine-Tuning and Customization
Fine-Tuning Options:
- OpenAI GPT-4-turbo fine-tuning (via JSONL datasets)
- LLaMA 3 fine-tuning using QLoRA + Hugging Face PEFT
Testing Prompt Variations:
- A/B test multiple system prompts
- Use GPT Evaluator to compare factual accuracy, helpfulness, tone
Custom Tools Integration:
- Define tool schemas (function calling)
- Add calculator, search, or custom logic via LangChain agents
Stage 6: User Management, Permissions, and Billing
Authentication & Authorization
- Integrate OAuth or SSO providers
- Role-based access: admin, team, enterprise
Usage-Based Billing
- Track tokens used via OpenAI’s response metadata
- Integrate with Stripe Metered Billing API
Usage Dashboard
- Tokens consumed per user/session
- Real-time costs and API throughput
Stage 7: DevOps, Hosting & CI/CD
Containerization
- Use Docker to containerize backend services
- Set up docker-compose for local dev
Cloud Deployment
- Frontend → Vercel / Cloudflare Pages
- Backend → AWS ECS, GCP Cloud Run, or Kubernetes
CI/CD Pipeline
- GitHub Actions or GitLab CI
- Auto-deploy on branch merges with staging and production separation
Monitoring & Logs
- Use Grafana + Prometheus for performance metrics
- Sentry / LogRocket for real-time frontend error logging
Stage 8: Analytics, Feedback, and Moderation
Analytics
- Log chat sessions, prompt usage, response time
- User satisfaction ratings (thumbs up/down)
Moderation Layer
- Use OpenAI’s moderation API or self-hosted content filter
- Flag or block offensive, harmful, or hallucinated responses
Feedback Loop
- Allow users to submit feedback on response quality
- Use responses to retrain or adjust prompts
Stage 9: Testing, QA, and Launch
Test Coverage
- Unit tests for core modules
- Integration tests for chat pipeline
- End-to-end tests using Cypress or Playwright
Pre-launch Checklist
- Load testing (simulate concurrent users)
- Token abuse detection
- API rate limiting
- Responsive design checks (mobile/tablet/desktop)
Stage 10: Post-Launch Maintenance and Iteration
Regular Maintenance
- Rotate OpenAI API keys and monitor quotas
Optimize slow LLM calls and latency spikes
Feature Iteration
- Add image input (multimodal GPT-4V)
- Train intent recognizers for command-style actions
- Build a plugin marketplace or prompt library
Summary of the Expanded Development Roadmap
Phase | Objective | Key Tools |
Planning | Define features and architecture | Miro, Lucidchart |
Frontend | Build dynamic chat UI | Next.js, Tailwind, Zustand |
Backend | API orchestration, LLM calls | FastAPI, Redis, PostgreSQL |
Memory & RAG | Contextual recall and embeddings | Pinecone, LangChain |
Fine-tuning | Specialize model behavior | QLoRA, PEFT |
Auth & Billing | Secure access and monetization | Clerk, Stripe |
DevOps | Deploy and maintain infrastructure | Docker, GitHub Actions |
Analytics & Moderation | Monitor and improve quality | Sentry, Prometheus |
QA & Testing | Ensure reliability | Cypress, PyTest |
Launch & Beyond | Iterate with confidence | Segment, Feature flags |
Building a Sustainable AI Product
Creating a ChatGPT-like clone in 2025 is fully achievable for skilled software teams. However, it requires more than just calling an API. You need a robust architecture, attention to security and UX, and an agile mindset to evolve with the fast-paced AI landscape.
Whether you’re building an internal chatbot, an AI-powered SaaS tool, or an industry-specific assistant, this guide provides the foundation, powered by the best of what 2025’s technology has to offer.
Need Help With Development or Architecture?
At O16 Labs, we have a team of AI engineers and full-stack developers who can help you build a scalable, secure, and feature-rich ChatGPT-like platform tailored to your business.
Related Blogs
Your Journey to Digital Transformation Starts Here
Perfect solutions take time to brew and come forth. Book a 10-minute call with our consultant to discuss what you seek and we’ll love sharing all the secrets. Hop on to the digital change bandwagon and ride your way to awesomeness.
We Are Located Here
San Antonio
1207 McCullough Ave.
San Antonio, TX 78212